When you have existing test suite containing jUni4 tests and you want to migrate slowly to jUnit5 to make use of new APIs and modern test engine, you may encounter some issues – not all tests will be visible in reports.
jUni4 and jUnit5 test classes
Let’s consider the following test classes, first one has @Test annotation from jUnit4, second one has @Test from jUni5:
class JUnit4Test{
@org.junit.Test
fun `it should fail - junit4`(){
throw AssertionError()
}
}
class JUnit5Test{
@org.junit.jupiter.api.Test
fun `it should fail - junit5`(){
throw AssertionError()
}
}
Adding jUnit5 to Android project
To your app/build.gradle add following configuration:
android {
//other configs
testOptions {
unitTests.all {
useJUnitPlatform()
}
}
}
dependencies {
// junit 5
testImplementation "org.junit.jupiter:junit-jupiter:5.8.0"
// junit 4
testImplementation "junit:junit:4.13.2"
}
in new Gradle versions there is no need to add junit5 plugin explicitly
Once you add useJunitPlatform() config line and run all tests, you will see following:
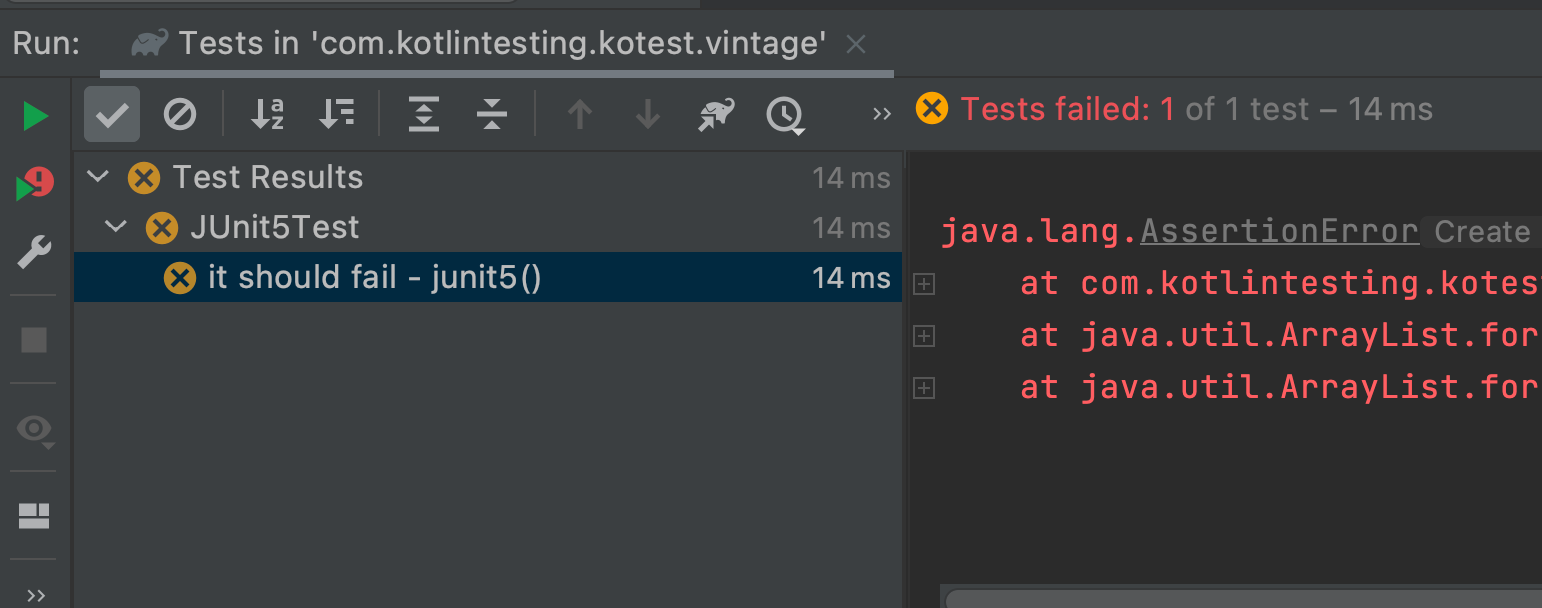
Adding junit vintage engine
To your app/build.gradle add junit-vintage-engine dependency:
// junit 5
testImplementation "org.junit.jupiter:junit-jupiter:5.8.0"
//junit 4
testImplementation "junit:junit:4.13.2"
// junit vintage engine
testImplementation "org.junit.vintage:junit-vintage-engine:5.8.0"
Once you add junit-vintage and run all tests once again, both jUnit4 and jUni5 tests will be visible for testing framework.
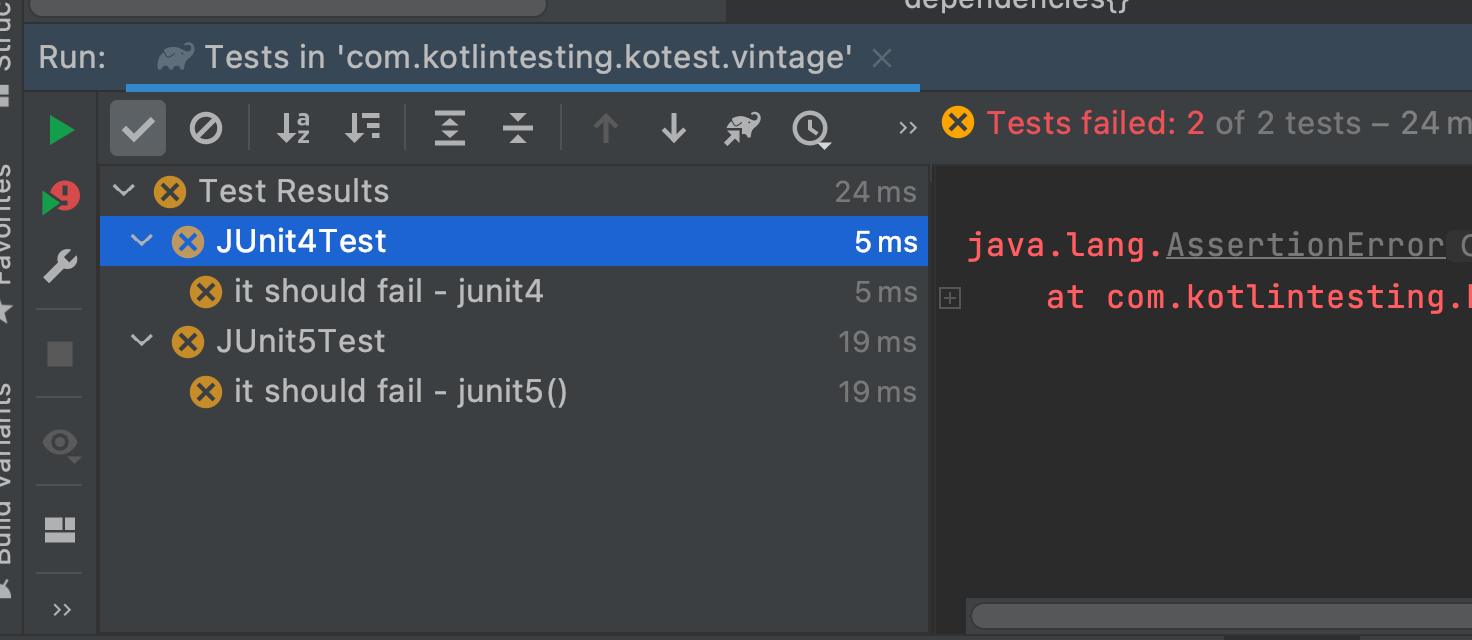
Remember – to verify that test framework is working, use tests with failing assertions.
More on test reports (what is green test, what is yellow and what is red) you can find here:
https://kotlintesting.com/pass-fail-crash/